Python Libraries: Essential Tools for Simplifying Programming
Updated on : 26 November, 2024, 10:00 IST
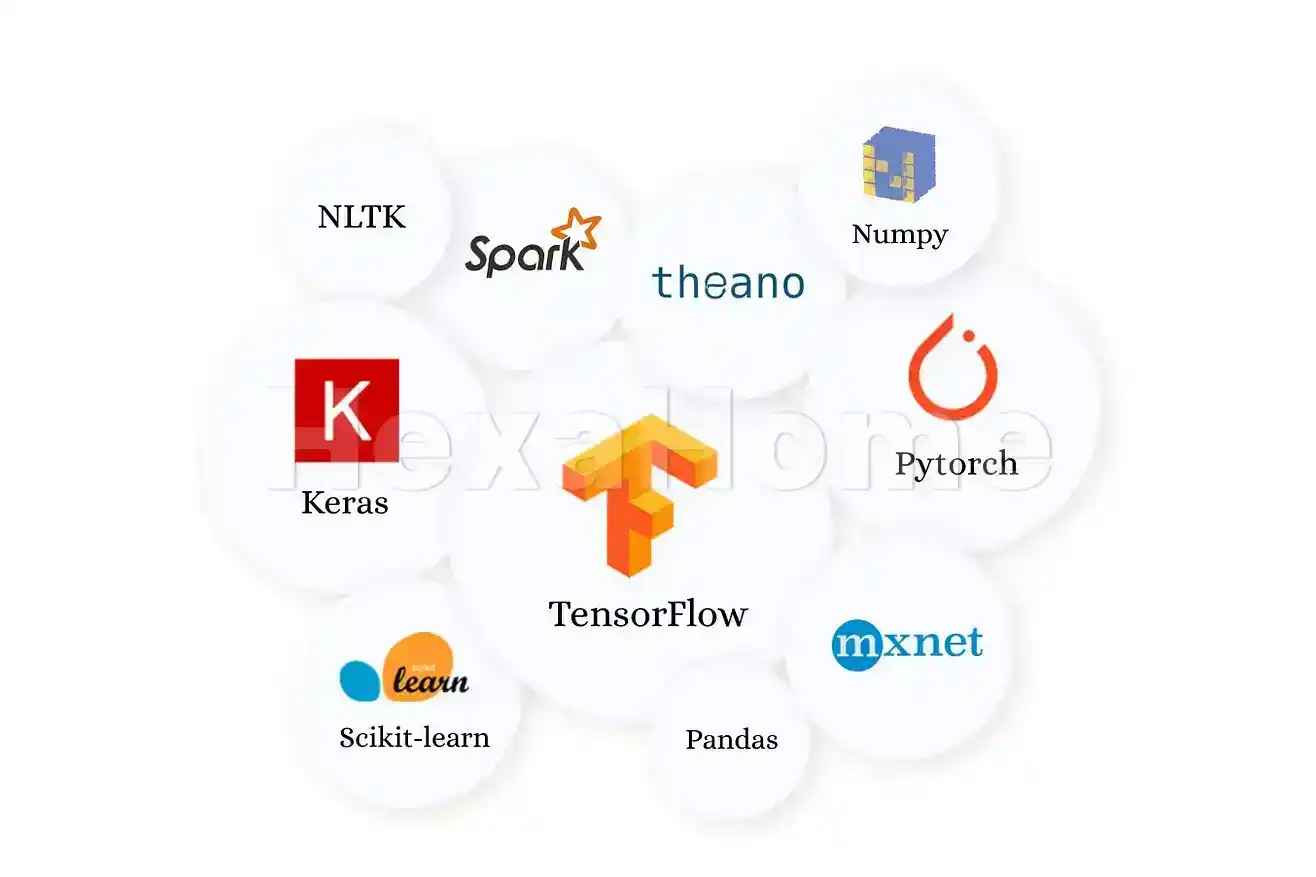
Image Source: freepik.com
Table Of Contents
What Are Python Libraries?
A Python library is essentially a collection of pre-written code modules that provide specific functionalities. Think of it as a toolbox filled with tools designed to help you complete various tasks efficiently. Instead of writing code from scratch for common operations—such as data manipulation, mathematical computations, or web development—developers can simply import these libraries and utilize their functionalities.
Why Are Python Libraries Important?
-
Efficiency: Libraries save developers time by providing ready-made solutions to common programming challenges. This allows them to focus on building features rather than writing boilerplate code.
-
Reusability: Code within libraries can be reused across multiple projects, promoting better practices in software development and reducing redundancy.
-
Quality and Reliability: Many libraries are developed and maintained by experienced programmers and communities, ensuring they undergo rigorous testing and refinement.
-
Community Support: Popular libraries often have active communities that provide support, documentation, and resources, making it easier for developers to learn and troubleshoot issues.
-
Extensibility: Libraries can often be customized or extended to meet specific project needs, allowing for flexibility in development.
The Top Python Libraries You Should Know
With thousands of libraries available, it can be overwhelming to choose which ones to learn. Here’s a curated list of some essential Python libraries that cater to various domains:
1. NumPy
NumPy is the foundation of numerical computing in Python. It provides support for large multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays. NumPy is widely used in scientific computing and data analysis due to its efficiency and speed.
Key Features:
- Powerful N-dimensional array object
- Broadcasting functions
- Tools for integrating C/C++ and Fortran code
Example Code Snippet
import numpy as np
# Create a 2D array (matrix)
array = np.array([[1, 2, 3], [4, 5, 6]])
# Perform element-wise addition
result = array + 10
print("Original Array:\n", array)
print("Array after Addition:\n", result)
2. Pandas
Built on top of NumPy, Pandas is an indispensable library for data manipulation and analysis. It introduces two primary data structures: Series (1D) and DataFrame (2D), which make it easy to handle structured data.
Key Features:
- Data cleaning and preparation
- Time series functionality
- Grouping and aggregation operations
Example Code Snippet
import pandas as pd
# Create a DataFrame
data = {
'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35],
'City': ['New York', 'Los Angeles', 'Chicago']
}
df = pd.DataFrame(data)
# Filter rows where Age is greater than 28
filtered_df = df[df['Age'] > 28]
print("Filtered DataFrame:\n", filtered_df)
3. Matplotlib
Matplotlib is a versatile plotting library that enables developers to create static, animated, and interactive visualizations in Python. It provides a MATLAB-like interface for creating high-quality graphs and charts.
Key Features:
- Extensive range of plot types (line plots, scatter plots, bar charts)
- Customizable visual styles
- Integration with Jupyter notebooks for interactive plotting
Example Code Snippet
import matplotlib.pyplot as plt
# Sample data
x = [1, 2, 3, 4]
y = [10, 15, 7, 10]
# Create a line plot
plt.plot(x, y)
plt.title('Sample Line Plot')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.grid(True)
plt.show()
4. Seaborn
Built on top of Matplotlib, Seaborn simplifies statistical data visualization by providing a high-level interface for drawing attractive graphics. It comes with several built-in themes and color palettes to enhance the aesthetics of your plots.
Key Features:
- Simplified syntax for complex visualizations
- Built-in themes for styling
- Support for categorical data visualization
Example Code Snippet
import seaborn as sns
import matplotlib.pyplot as plt
# Load an example dataset
tips = sns.load_dataset('tips')
# Create a scatter plot with regression line
sns.regplot(x='total_bill', y='tip', data=tips)
plt.title('Total Bill vs Tip')
plt.show()
5. SciPy
SciPy builds on NumPy by adding a collection of algorithms and mathematical tools for scientific computing. It includes modules for optimization, integration, interpolation, eigenvalue problems, algebraic equations, and more.
Key Features:
- Advanced mathematical functions
- Integration with NumPy arrays
- Optimization algorithms
Example Code Snippet
from scipy import optimize
# Define a simple quadratic function
def func(x):
return x**2 + 5*x + 6
# Find the minimum value using SciPy's optimize module
result = optimize.minimize(func, x0=0)
print("Minimum value occurs at x =", result.x[0])
print("Minimum value is =", func(result.x[0]))
6. TensorFlow
TensorFlow is an open-source library developed by Google for numerical computation using data flow graphs. It is widely used in machine learning applications, especially deep learning models.
Key Features:
- Flexible architecture allowing deployment across various platforms (CPUs, GPUs)
- High-level APIs like Keras for easy model building
- Extensive community support and documentation
Example Code Snippet
import tensorflow as tf
# Define a simple sequential model using Keras API
model = tf.keras.Sequential([
tf.keras.layers.Dense(10, activation='relu', input_shape=(None, 1)),
tf.keras.layers.Dense(1)
])
model.compile(optimizer='adam', loss='mean_squared_error')
# Generate some sample data for training
import numpy as np
x_train = np.random.rand(1000).astype(np.float32)
y_train = x_train * 2 + np.random.normal(0, 0.1, size=x_train.shape)
# Train the model
model.fit(x_train.reshape(-1, 1), y_train.reshape(-1, 1), epochs=10)
7. PyTorch
Developed by Facebook’s AI Research lab, PyTorch is another popular open-source machine learning library that emphasizes flexibility and speed. It offers dynamic computational graphs that make it easier to build complex models.
Key Features:
- Intuitive interface
- Strong GPU acceleration capabilities
- Extensive support for neural networks
Example Code Snippet
import torch.nn as nn
import torch.optim as optim
import torch
# Define a simple feedforward neural network model
class SimpleNN(nn.Module):
def __init__(self):
super(SimpleNN, self).__init__()
self.fc1 = nn.Linear(1, 10)
self.fc2 = nn.Linear(10, 1)
def forward(self, x):
x = torch.relu(self.fc1(x))
return self.fc2(x)
model = SimpleNN()
criterion = nn.MSELoss()
optimizer = optim.Adam(model.parameters(), lr=0.01)
# Generate sample data for training
x_train = torch.rand(1000).view(-1, 1)
y_train = x_train * 2 + torch.randn(1000).view(-1, 1) * 0.1
# Training loop
for epoch in range(100):
model.train()
optimizer.zero_grad()
outputs = model(x_train)
loss = criterion(outputs, y_train)
loss.backward()
optimizer.step()
print("Training complete.")
8. Scikit-Learn
Scikit-Learn is one of the most widely used machine learning libraries in Python. It provides simple and efficient tools for predictive data analysis built on NumPy, SciPy, and Matplotlib.
Key Features:
- Easy-to-use API for implementing various algorithms (classification, regression)
- Tools for model selection and evaluation
- Comprehensive documentation with examples
Example Code Snippet
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
from sklearn.datasets import make_regression
# Generate sample regression data
X, y = make_regression(n_samples=1000, n_features=1, noise=0.1)
# Split the dataset into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y)
# Create a linear regression model
model = LinearRegression()
model.fit(X_train, y_train)
# Predict on the test set
predictions = model.predict(X_test)
print("Model Coefficients:", model.coef_)
9. Flask
Flask is a lightweight web framework that allows developers to build web applications quickly using Python. Its simplicity makes it an excellent choice for small projects or microservices.
Key Features:
- Minimalistic design with modular components
- Built-in development server
- Easy integration with databases through extensions
Example Code Snippet
from flask import Flask
app = Flask(__name__)
@app.route('/')
def home():
return "Hello World! Welcome to my Flask app."
if __name__ == '__main__':
app.run(debug=True)
10. Django
Django is a high-level web framework that encourages rapid development and clean design principles. It comes with many built-in features such as authentication, URL routing, ORM (Object Relational Mapping), and more.
Key Features:
- Robust security features
- Scalable architecture suitable for large applications
- Comprehensive documentation and community support
Example Code Snippet
from django.http import HttpResponse
from django.urls import path
def home(request):
return HttpResponse("Hello World! Welcome to my Django app.")
urlpatterns = [
path('', home),
]
Best Practices for Using Python Libraries
To maximize the benefits of Python libraries in your projects, consider these best practices:
-
Thorough Documentation: Always refer to the library's documentation to understand its functionalities thoroughly before implementation.
-
Compatibility Checks: Ensure that the library version you are using aligns with your version of Python to avoid compatibility issues.
-
Testing Before Production Use: Experiment with libraries in a controlled environment before deploying them in production.
-
Use Virtual Environments: Isolate different project dependencies using virtual environments to prevent conflicts between packages.
-
Version Control: Keep track of changes in your libraries using version control systems like Git.
-
Maintain Code Readability: Follow consistent coding standards to ensure your code remains readable and maintainable over time.
Conclusion
Python's extensive library ecosystem significantly enhances its capabilities as a programming language making it easier for developers to build robust applications efficiently. By leveraging these powerful tools—whether you're working on data analysis machine learning web development or scientific computing—you can save time while ensuring high-quality results.
As you continue your journey in Python programming explore different libraries based on your project requirements experiment with their functionalities The right library can transform your coding experience from tedious to exhilarating—unlocking new possibilities in your development endeavors! Happy coding!