Java Applet Programming Quick Guide
Updated on : 19 MAY 2025
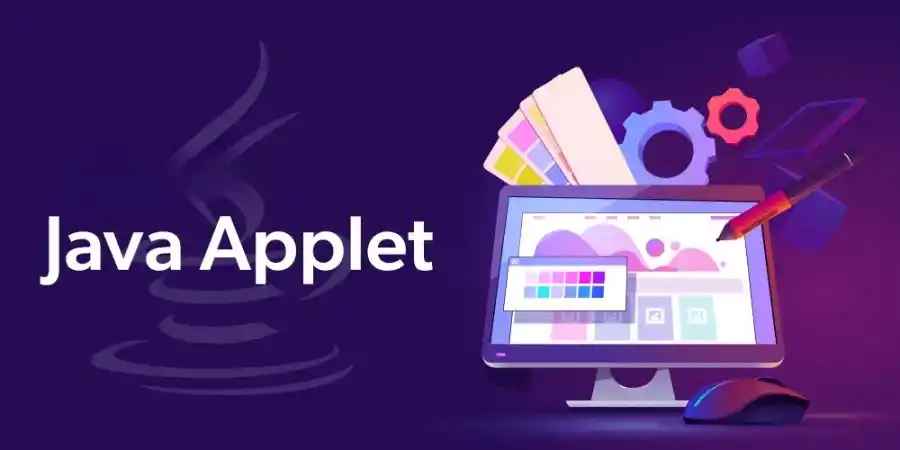
Image Source: google.com
Table Of Contents
- 1. Introduction
- 2. Java Applet Code Examples with Output
- 3. Stages in the Java Applet Life Cycle
- 4. Initialization to Termination Process
- 5. Types of Java Applets
- 6. Creating a Simple Java Applet
- 7. Java Applet Architecture
- 8. Applet Execution Flow
- 9. Initialization Methods in Applets
- 10. Starting and Stopping an Applet
- 11. Rendering and Cleanup Processes
- 12. Other Useful Applet Methods
- 13. FAQs
Table Of Contents
Introduction
Java Applet Programming Quick Guide Dive into Java applets—tiny, powerful programs that once brought life to web pages with interactive magic! Running inside your browser, they used Java to create dynamic content. Though now rare, applets paved the way for todays web experiences!
Java Applet Code Examples with Output
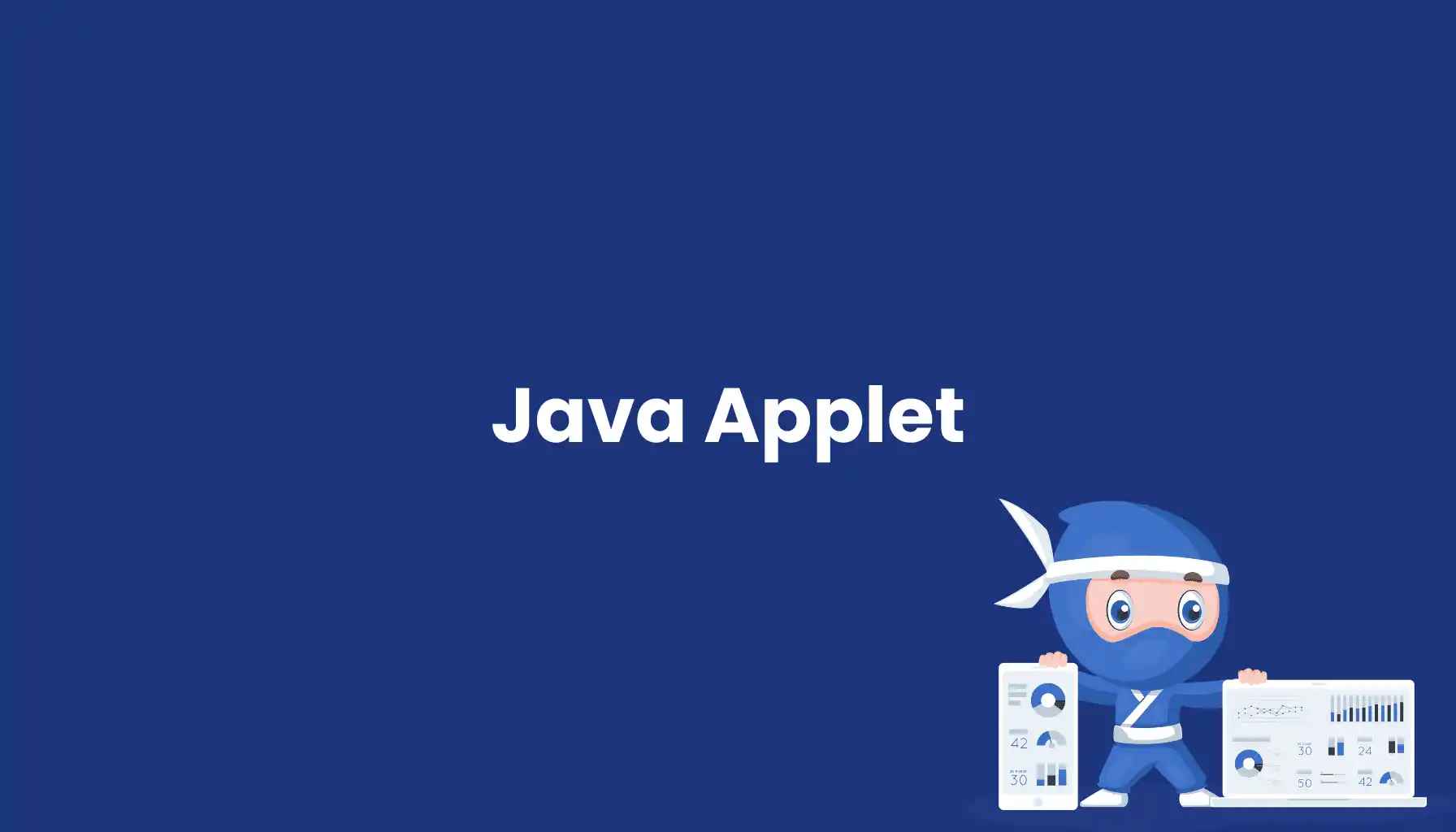
Image Source: google
This section explains how to create and run a simple Java applet, including a code example and the steps required to execute it.
-
Example: Simple Java Applet to Display a Message
-
Demonstrates a basic Java applet program.
-
Typically uses the
Applet
class and overrides methods likepaint(Graphics g)
to display messages. -
Example code may include:
import java.applet.Applet; import java.awt.Graphics; public class HelloApplet extends Applet { public void paint(Graphics g) { g.drawString("Hello, Java Applet!", 50, 50); } }
-
-
Steps to Run the Java Applet
-
Step 1: Write the code in a
.java
file and compile it usingjavac
. -
Step 2: Create an HTML file to embed the applet using the
<applet>
tag (or<object>
/<embed>
in newer versions). -
Step 3: Use an applet viewer or compatible browser to run the HTML file.
-
Example HTML snippet:
<applet code="HelloApplet.class" width="300" height="200"> </applet>
-
-
Note
- Java applet support is deprecated in modern browsers.
- Applets can still be tested using the
appletviewer
tool provided by JDK.
Stages in the Java Applet Life Cycle
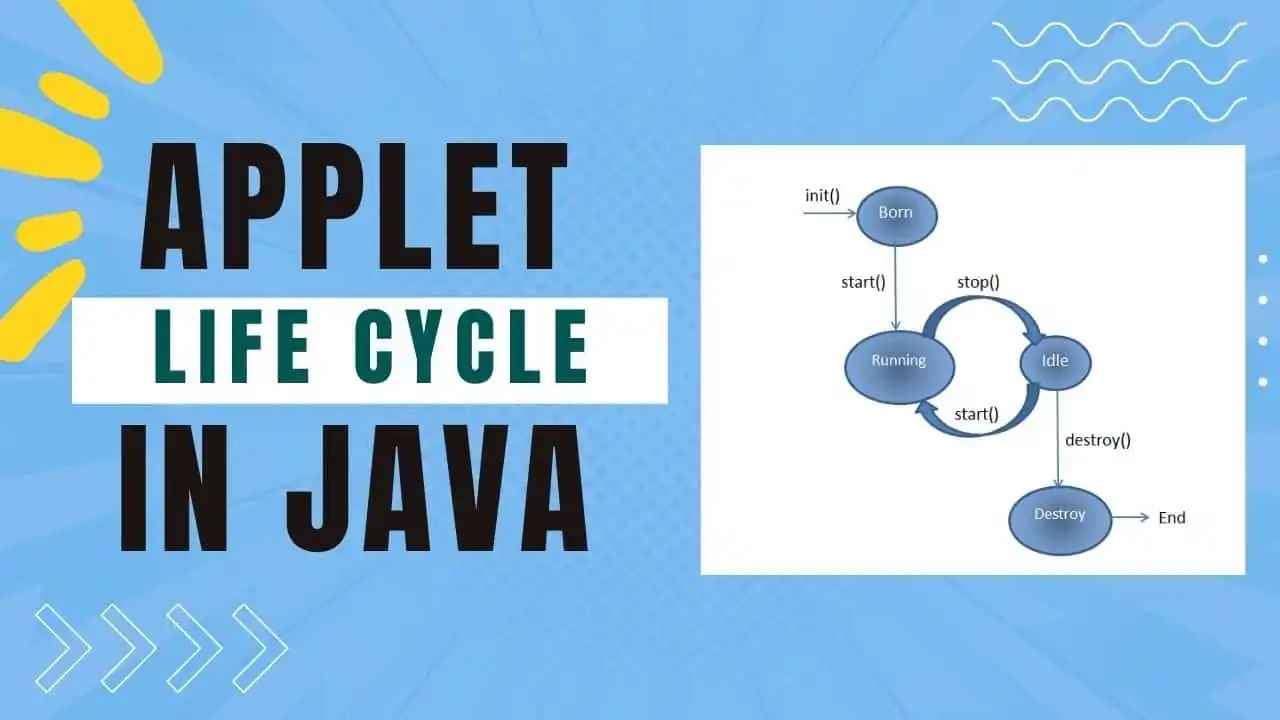
Image Source: google
🔄 Life Cycle Stage | 📋 Description |
---|---|
Initialization (`init()`) | Called once when the applet is first loaded; used for setup tasks. |
Start (`start()`) | Invoked after `init()` and every time the applet becomes active or visible. |
Paint (`paint(Graphics g)`) | Handles drawing on the screen; called whenever the applet needs to be redrawn. |
Stop (`stop()`) | Called when the applet is no longer active or moves out of view. |
Destroy (`destroy()`) | Executed just before the applet is removed from memory; used to release resources. |
Initialization to Termination Process
📘 Java Applet Programming Quick Guide
This process describes the five main stages that a Java applet goes through from beginning to end.
- 🟢
init()
– Initialization
- Called once when the applet is loaded.
- Used for setting up initial configurations.
- ▶️
start()
– Start
- Called after
init()
and every time the applet becomes active. - Ideal for starting animations or threads.
- 🎨
paint(Graphics g)
– Paint
- Used to draw content on the applet window.
- Automatically called after
start()
or when refreshing.
- ⏸️
stop()
– Stop
- Called when the applet is not active or minimized.
- Stops ongoing activities like threads.
- ❌
destroy()
– Termination
- Final stage before the applet is removed from memory.
- Used for releasing resources or cleanup.
Java Applet Programming Quick Guide helps you understand how applets are structured and executed from start to finish.
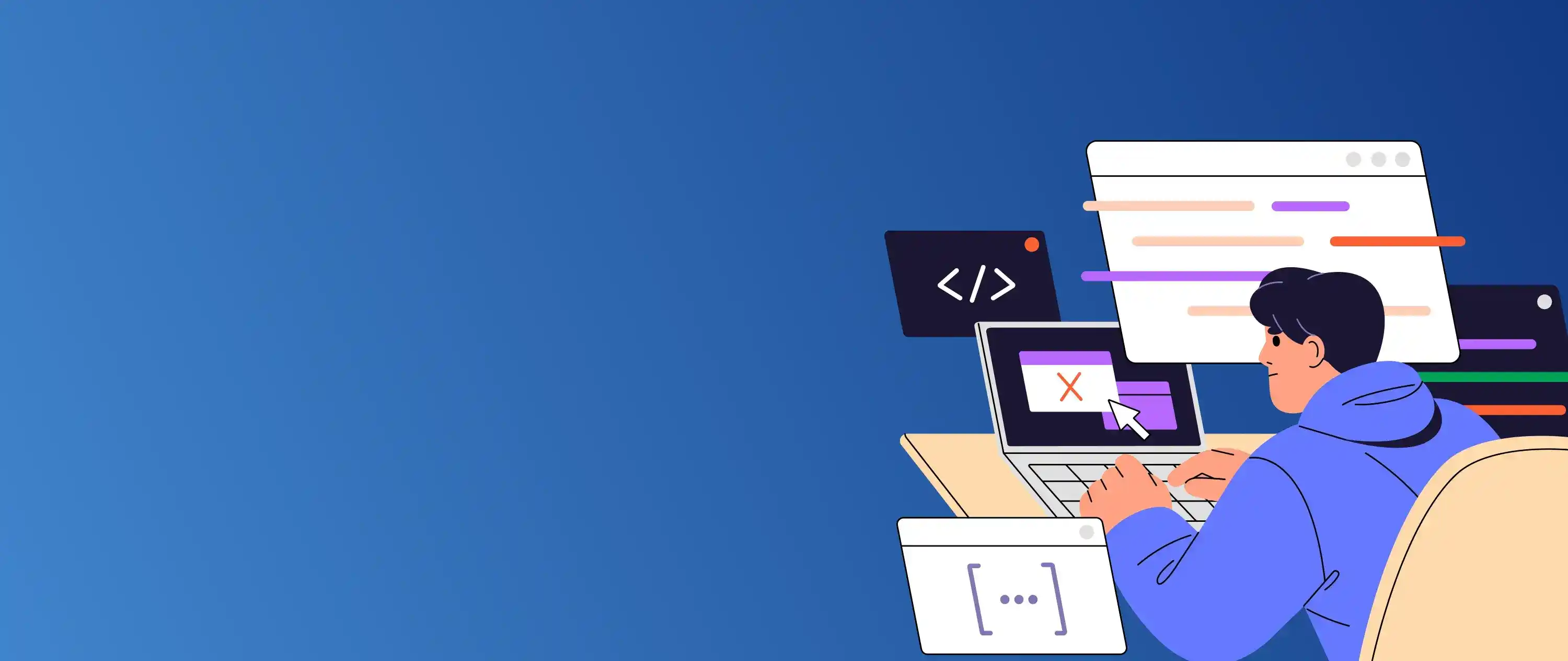
Need help for Hire Java Developers?
Types of Java Applets
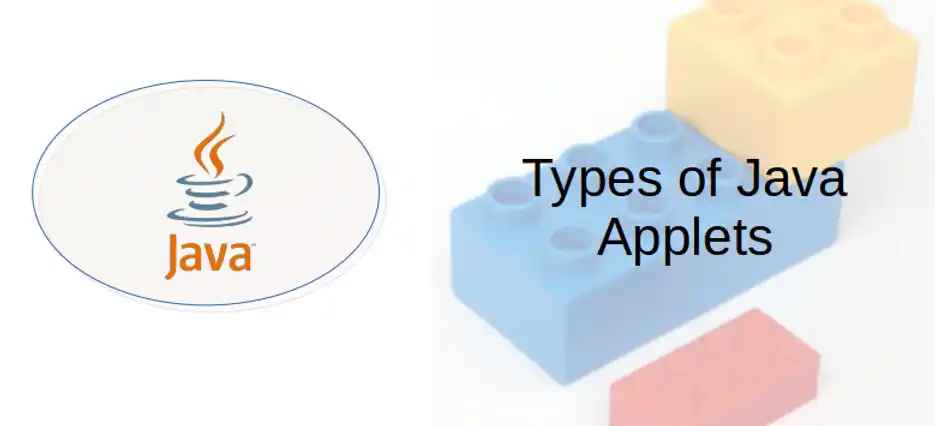
Image Source: google
🔠 Type | 📋 Description |
---|---|
Local Applet | Stored on the local system and loaded using the file system path. Does not require internet. |
Remote Applet | Stored on a remote server and accessed via a URL over the internet using a web browser. |
Creating a Simple Java Applet
📘 Java Applet Programming Quick Guide
This section explains the steps to create, compile, and run a basic Java applet.
- 📝 Write the Applet Code
- Create a
.java
file usingApplet
class. - Override methods like
init()
orpaint(Graphics g)
.
- 🧮 Compile the Code
- Use
javac
to compile the source file. - Example:
javac HelloApplet.java
- 🌐 Create an HTML File
-
Embed the compiled
.class
file using the<applet>
tag. -
Example:
<applet code="HelloApplet.class" width="300" height="200"></applet>
- 🔍 Run the Applet
- Use
appletviewer
or a compatible browser to test. - Example:
appletviewer HelloApplet.html
📘 Java Applet Programming Quick Guide ensures beginners learn each step clearly—from writing code to running an applet smoothly.
You Might Also Like
Java Applet Architecture
🏗️ Component | 📋 Description |
---|---|
Applet Class | Extends the Applet or JApplet class; contains core lifecycle methods. |
AWT (Abstract Window Toolkit) | Used for creating GUI elements and handling graphics through `paint(Graphics g)`. |
Life Cycle Methods | Controls the applet's stages: `init()`, `start()`, `stop()`, and `destroy()`. |
Browser/Applet Viewer | Hosts and runs the applet, providing the necessary runtime environment. |
HTML File | Loads the applet using the `<applet>` tag and serves as the front-end container. |
Applet Execution Flow
📘 Java Applet Programming Quick Guide
Applet execution flow refers to the sequence of method calls that control the applet’s behavior from loading to termination.
- 🔁 Load Phase
- Browser or applet viewer loads the applet class.
- Triggers the execution of the
init()
method.
- ▶️ Start Phase
- After initialization, the
start()
method is called. - Begins execution like animations or background tasks.
- 🖌️ Paint Phase
- The
paint(Graphics g)
method is called to display output. - Automatically invoked when the applet needs redrawing.
- ⏸️ Stop Phase
- When the applet is paused (e.g., window minimized),
stop()
is called. - Pauses any ongoing processes.
- ❌ Destroy Phase
- Before closing,
destroy()
is invoked to release resources.
📘 Java Applet Programming Quick Guide helps you understand this flow clearly so you can build efficient and responsive applets.
Initialization Methods in Applets
🛠️ Method | 📋 Purpose |
---|---|
init() | Called once when the applet is first loaded. Used for one-time initialization like setting variables or loading resources. |
start() | Called after `init()` and every time the applet becomes active. Used to start or resume tasks such as animations. |
paint(Graphics g) | Called after `start()` and whenever the applet needs to redraw its content. Used for displaying output. |
stop() | Called when the applet is not visible or inactive. Used to pause ongoing processes. |
destroy() | Called before the applet is removed from memory. Used to release resources and perform cleanup. |
Starting and Stopping an Applet
📘 Java Applet Programming Quick Guide
This section explains how an applet begins execution and how it pauses or stops based on user interaction or browser events.
- ▶️
start()
– Starting the Applet
- Invoked after
init()
or when the applet becomes active. - Used to start animations, threads, or media playback.
- ⏸️
stop()
– Stopping the Applet
- Called when the user navigates away or minimizes the browser window.
- Used to pause tasks, save data, or free up resources.
- ♻️ Repeated Calls
start()
andstop()
may be called multiple times during the applet's life.- Helps manage the applet's behavior based on visibility.
📘 Java Applet Programming Quick Guide ensures a clear understanding of how to manage applet activity efficiently for smooth user interaction.
Rendering and Cleanup Processes
🔧 Process | 📋 Description |
---|---|
Rendering (`paint(Graphics g)`) | Handles all visual output of the applet. Automatically called to draw or refresh the screen content. |
Repainting (`repaint()`) | Requests the applet to redraw itself by indirectly calling `update()` and then `paint()`. |
Cleanup (`stop()`) | Temporarily halts tasks when the applet is inactive or not visible. |
Final Cleanup (`destroy()`) | Called before the applet is removed from memory. Used to release resources and close connections. |
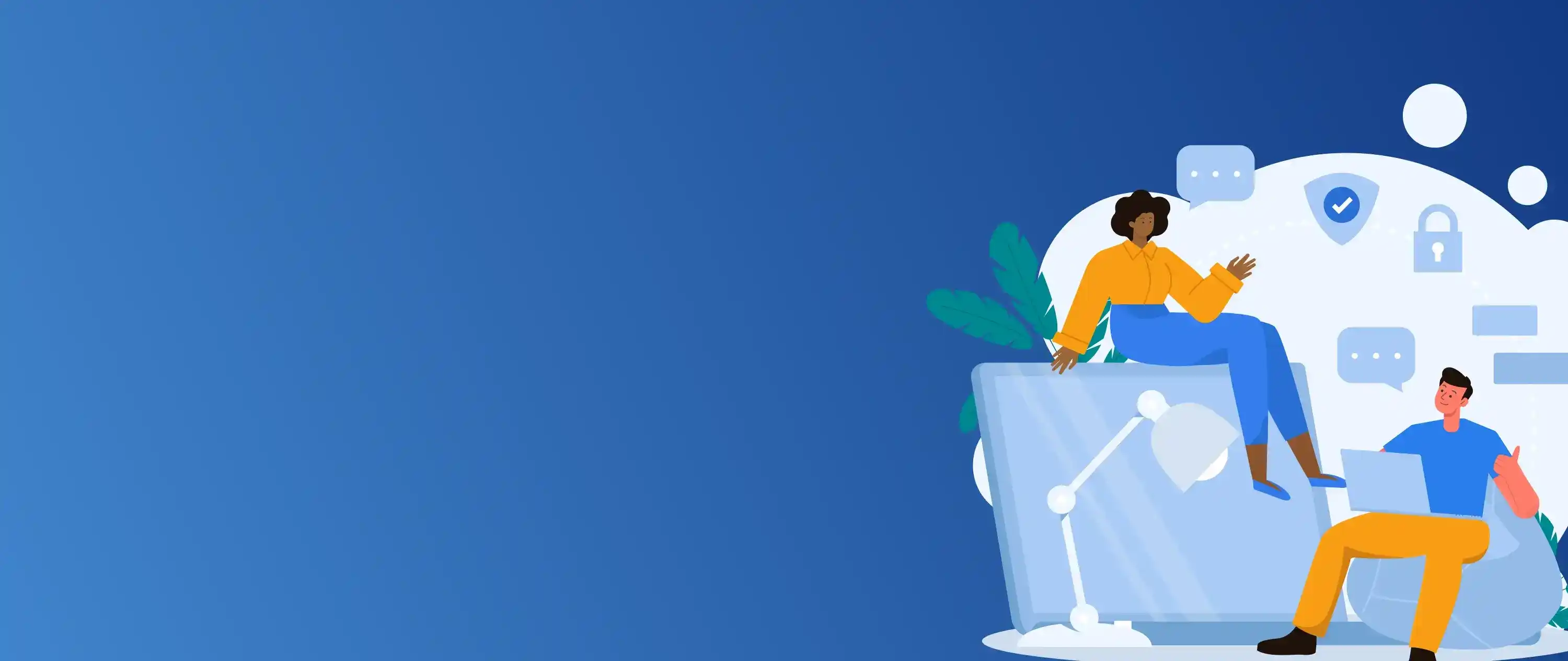
Do You Want to Hire Expert Java Developers?
Other Useful Applet Methods
Java Applet Programming Quick Guide
Besides the main applet methods (init()
, start()
, stop()
, destroy()
), these methods are also helpful:
-
getCodeBase()
-
Gets the URL where the applet code is stored.
-
getDocumentBase()
-
Gets the URL of the web page showing the applet.
-
getParameter(String name)
-
Gets a value from the applet’s HTML parameters.
-
getWidth() and getHeight()
-
Get the applet’s current width and height.
-
showStatus(String message)
-
Shows a message in the browser’s status bar.
-
resize(int width, int height)
-
Changes the size of the applet window.
FAQs
Q.1. What is a Java applet?
A : A small Java program that runs in a web browser.Q.2. How is an applet different from a normal Java program?
A : It runs in a browser and has no main method.Q.3. What are the main applet methods?
A : init(),
start(),
stop(),
destroy()`.Q.4. How do you run an applet?
A : Put it inside an HTML page using<applet>
tag.Q.5. Can applets access your computer files?
A : No, for security reasons.Q.6. What does getParameter()
do?
Q.7. Is Java applet still used?
A : No, it’s mostly outdated now.Q.8. What is showStatus()
for?