Advanced Java Programming Concepts
Updated on : 20 MAY 2025
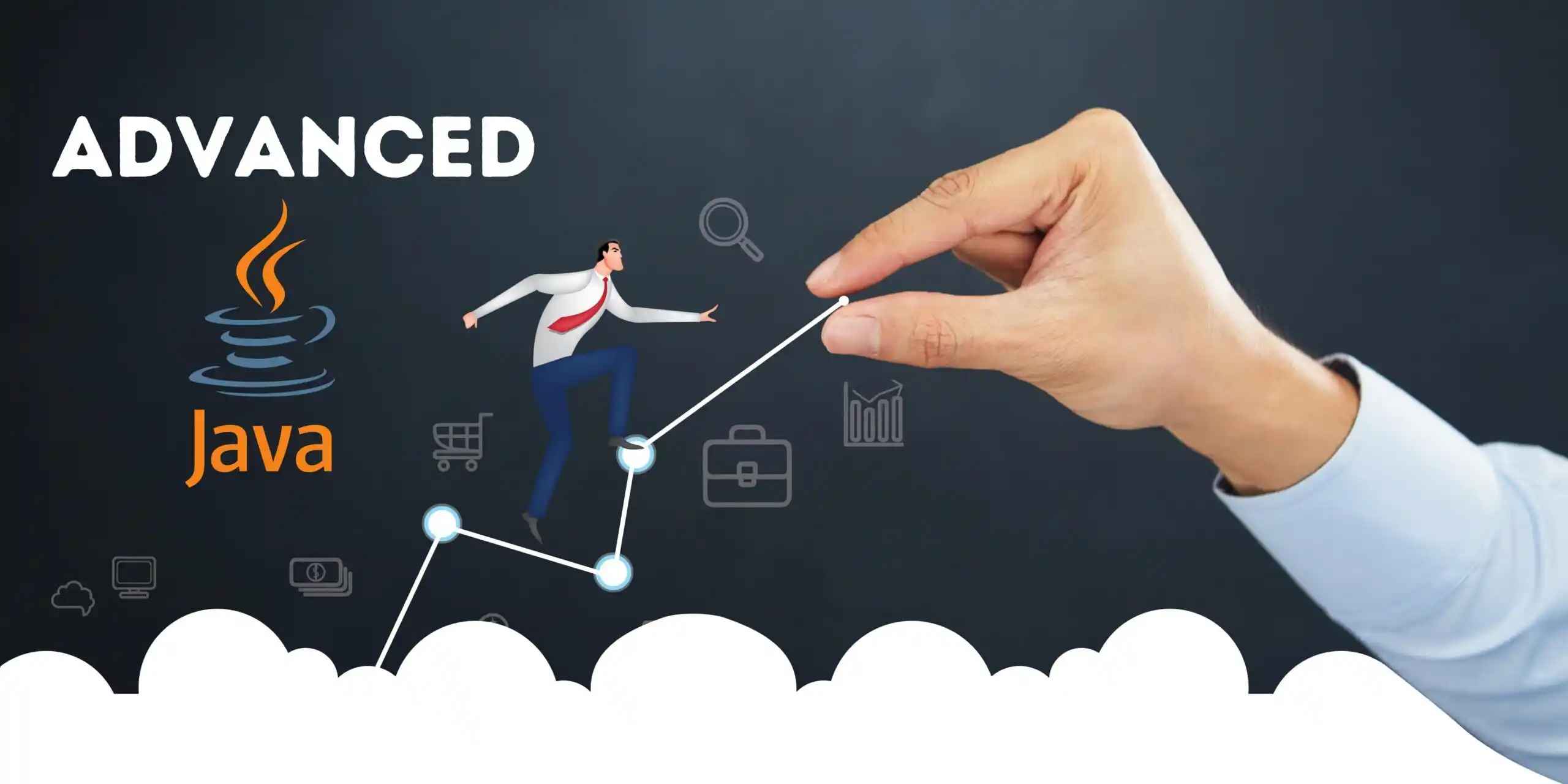
Image Source: google.com
Table Of Contents
- 1. Introduction
- 2. What is Advanced Java
- 3. Java Database Connectivity
- 4. Java Servlets and JSP
- 5. Model-View-Controller Architecture
- 6. Session Management in Web Applications
- 7. Enterprise JavaBeans
- 8. Spring Framework Essentials
- 9. Hibernate and ORM
- 10. Java Messaging Service
- 11. RESTful Web Services with JAX-RS
- 12. Java Authentication and Authorization
- 13. Design Patterns in Advanced Java
- 14. FAQs
Table Of Contents
Introduction
Advanced Java Programming Concepts open the door to dynamic web apps and enterprise solutions. From JDBC to Servlets and JSP, it adds power beyond core Java. Perfect for those ready to level up, Advanced Java Programming Concepts bring real-world development to life with speed, scalability, and smart features.
What is Advanced Java
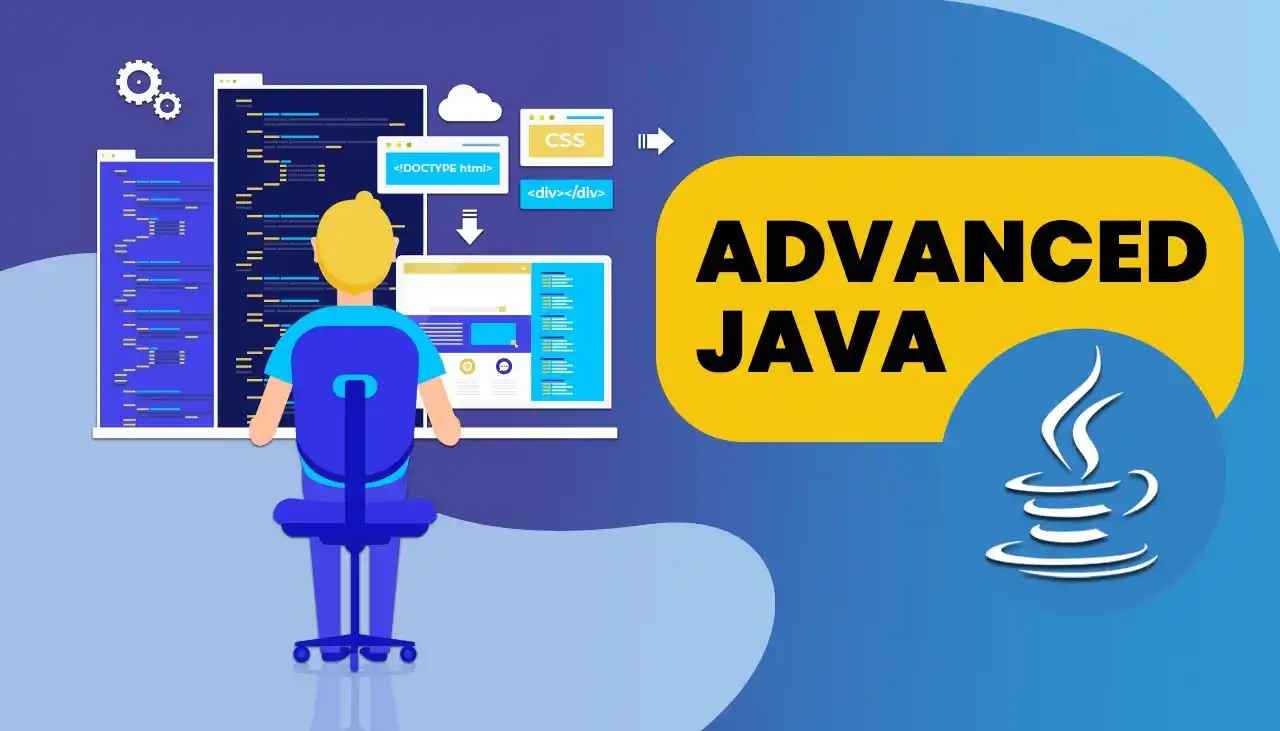
Image Source: google
Advanced Java refers to the next level of Java programming used for developing web-based, networked, and enterprise-level applications. It builds on core Java by adding more powerful tools and technologies.
🔑 Key Points:
-
📘 Definition: Advanced Java focuses on specialized areas like web development, networking, and database handling.
-
🛠️ Technologies Included: It includes Servlets, JSP (JavaServer Pages), JDBC (Java Database Connectivity), RMI, and more.
-
🌐 Use Cases: Used for creating dynamic websites, enterprise applications, and client-server architecture.
-
🚀 Importance: Advanced Java Programming Concepts are essential for building large-scale, robust, and secure applications.
-
💻 Real-world Application: Advanced Java Programming Concepts help in developing real-time web apps, online portals, and backend systems.
Simple, powerful, and practical—Advanced Java takes programming to the professional level. 💼✨
Java Database Connectivity
🔌 JDBC Component | 📋 Description |
---|---|
DriverManager | Manages a list of database drivers and establishes a connection between Java application and database. |
Connection | Represents a session with a specific database; used to execute SQL statements. |
Statement | Used to execute static SQL queries without parameters. |
PreparedStatement | Executes precompiled SQL queries with input parameters; safer and faster than Statement. |
ResultSet | Represents the result of a SQL query; allows navigation and reading of returned data. |
SQLException | Handles database access errors and provides details about exceptions during JDBC operations. |
Java Servlets and JSP
🧩 Component | Servlet | JSP |
---|---|---|
Purpose | Handles HTTP requests and responses; controls backend logic. | Used to create dynamic web pages by embedding Java code in HTML. |
Code Type | Pure Java code inside Java classes. | HTML with embedded Java code (scriptlets) or Expression Language. |
Compilation | Compiled as Java bytecode (.class files). | Translated into Servlets by the server at runtime. |
Configuration | Configured via deployment descriptor (web.xml) or annotations. | Configured as JSP files, automatically managed by the container. |
Use Case | Processing requests, controlling business logic, session management. | Presentation layer to display data to users. |
Communication | Uses ServletConfig and ServletContext for config and context. | Uses directives and EL for data binding and control. |
Ease of Use | More complex, requires Java programming skills. | Easier for designers familiar with HTML. |
Model-View-Controller Architecture
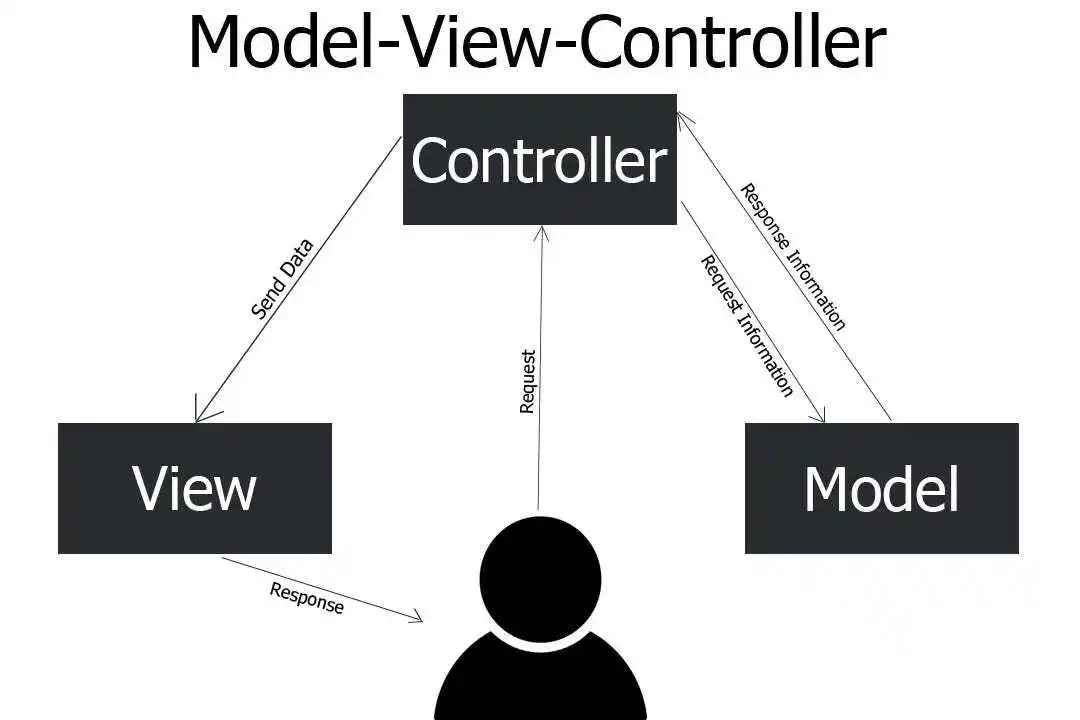
Image Source: google
Model-View-Controller (MVC) is a design pattern used in software development to separate application logic into three interconnected components, making code more organized, maintainable, and scalable. It plays a key role in Advanced Java Programming Concepts.
-
Model (🗄️ Data Layer): Manages the data, logic, and rules of the application. It interacts with the database and performs data operations.
-
View (🖥️ Presentation Layer): Displays the data provided by the model in a user-friendly format. JSP (JavaServer Pages) are commonly used in this layer.
-
Controller (🎮 Logic Layer): Handles user input, updates the model, and decides which view to display. Servlets typically act as controllers in Advanced Java Programming Concepts.
MVC architecture promotes separation of concerns, making development more efficient and testing easier. It is a foundational part of web frameworks in Advanced Java Programming Concepts.
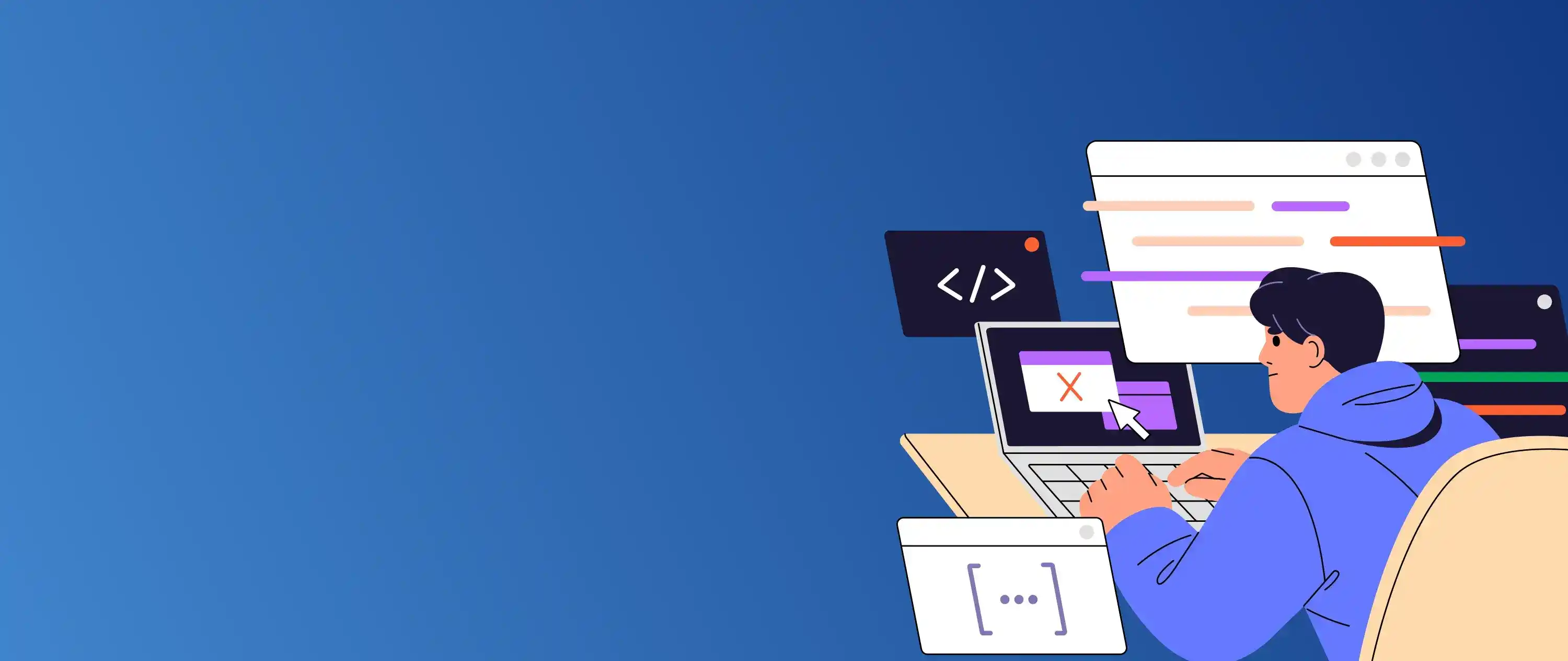
Need help for Hire Java Developers?
Session Management in Web Applications
🛠️ Technique | 📋 Description |
---|---|
Cookies | Small pieces of data stored on the client side to identify users and manage sessions. |
URL Rewriting | Appends session ID to the URL when cookies are disabled; maintains session tracking. |
Hidden Form Fields | Stores session information in hidden HTML form fields submitted with each request. |
HttpSession | A server-side object in Java (Servlets) used to store user data between requests during a session. |
Token-Based Authentication | Uses secure tokens (like JWT) to manage and validate user sessions across requests. |
You Might Also Like
Enterprise JavaBeans
📦 EJB Type | 📋 Description |
---|---|
Session Bean | Performs business logic for a client. Can be stateless, stateful, or singleton. |
Stateless Session Bean | Does not maintain any conversational state with the client between requests. |
Stateful Session Bean | Maintains state across multiple method calls and transactions from the same client. |
Singleton Session Bean | A single shared instance used for shared tasks like caching or resource initialization. |
Message-Driven Bean | Handles asynchronous messages from a queue or topic, typically used in JMS. |
Entity Bean (Deprecated) | Was used to represent persistent data stored in a database; now replaced by JPA. |
Spring Framework Essentials
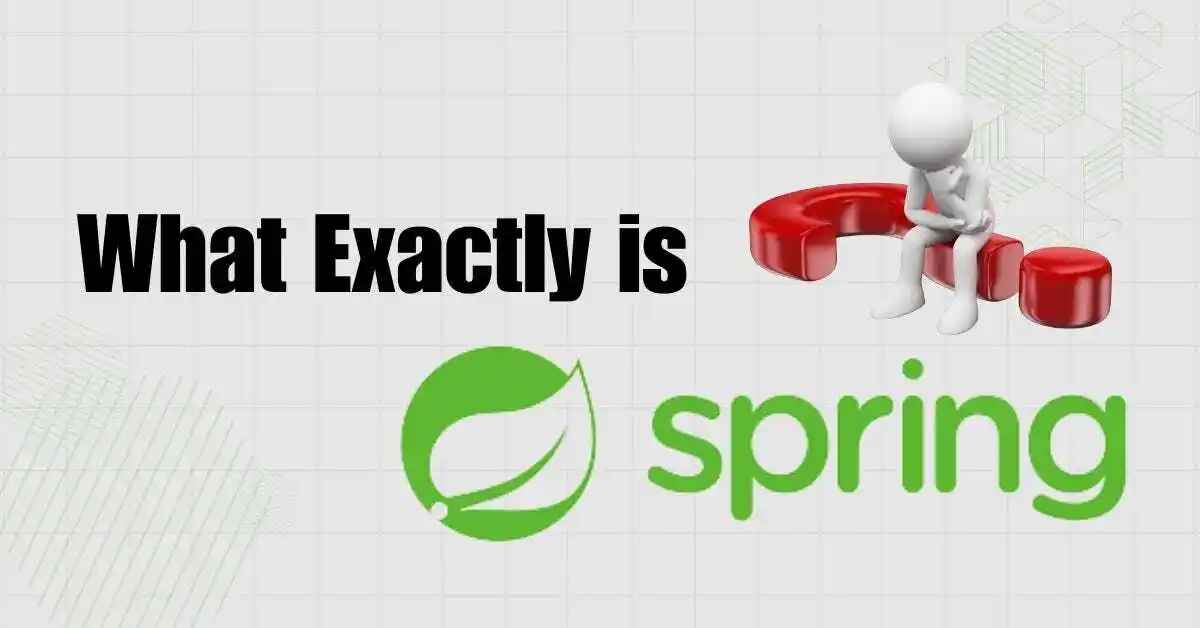
Image Source: google
The Spring Framework is a powerful, lightweight framework used in Java development to build enterprise-grade applications with ease and flexibility. It is a core part of Advanced Java Programming Concepts and supports various features like dependency injection, AOP, and MVC architecture.
-
Dependency Injection (DI) 🧩 Simplifies object creation and management by injecting dependencies at runtime, improving testability and code flexibility.
-
Aspect-Oriented Programming (AOP) 🎯 Enables modularizing cross-cutting concerns like logging, security, and transactions.
-
Spring MVC 🌐 A framework for building robust web applications using the Model-View-Controller pattern.
-
Spring Boot 🚀 Simplifies Spring setup and development with embedded servers, auto-configuration, and minimal XML configuration.
-
Data Access (Spring JDBC & JPA) 🗃️ Makes it easy to work with relational databases using templates and ORM tools.
The Spring Framework enhances productivity and reduces boilerplate code, making it a cornerstone of Advanced Java Programming Concepts for modern application development.
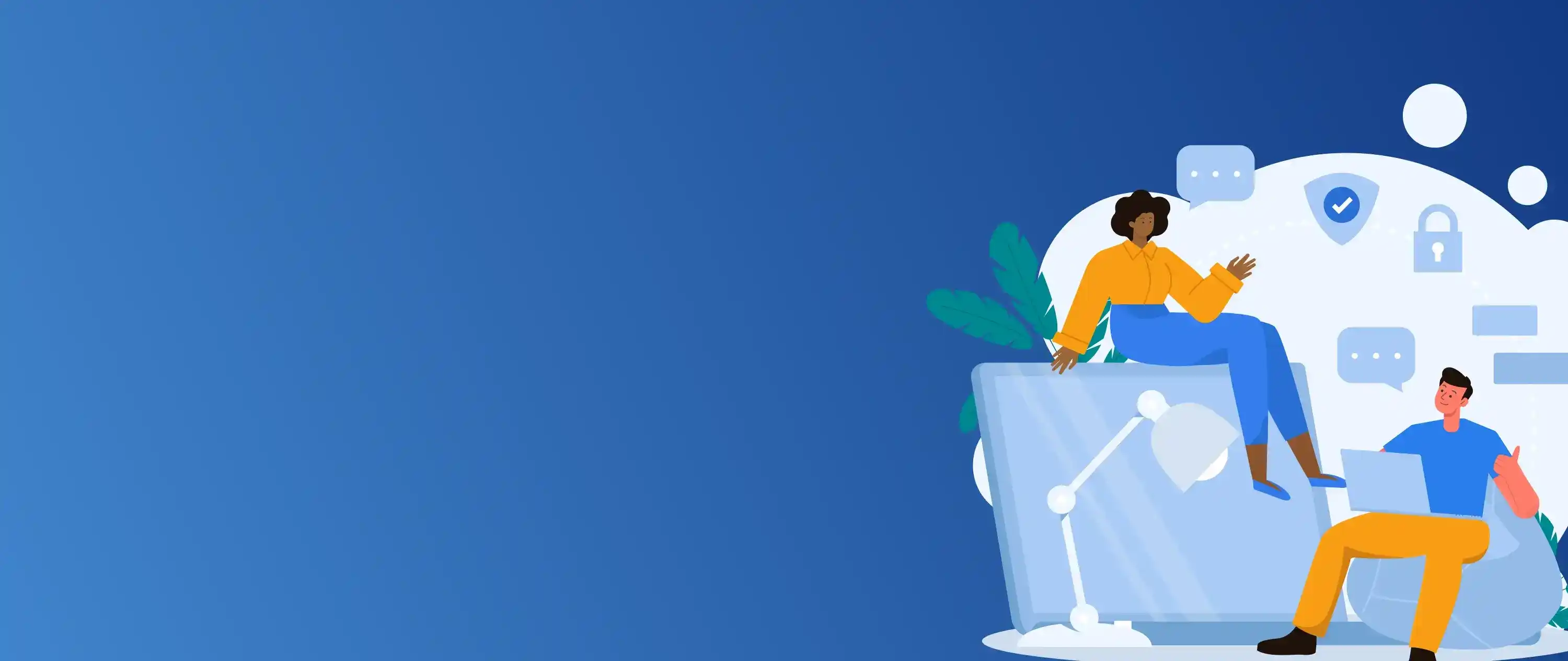
Do You Want to Hire Expert Java Developers?
Hibernate and ORM
🧩 Concept | Definition | Role in Hibernate/ORM |
---|---|---|
Hibernate | A Java framework for ORM. | Implements ORM to map Java objects to database tables and manages database operations. |
ORM (Object-Relational Mapping) | Technique connecting object-oriented code to relational databases. | Abstracts database interactions to work with objects rather than SQL queries. |
SessionFactory | Factory for creating sessions. | Manages and configures database connections and sessions in Hibernate. |
Session | Single-threaded unit of work with DB. | Handles CRUD operations and queries within a transaction scope. |
Configuration | Setup for Hibernate. | Loads settings like DB URL, driver, dialect, and mappings. |
Hibernate Query Language (HQL) | Object-oriented query language. | Allows querying database using entity names and properties instead of tables and columns. |
Entity | Java class mapped to DB table. | Represents persistent data objects managed by Hibernate. |
Java Messaging Service
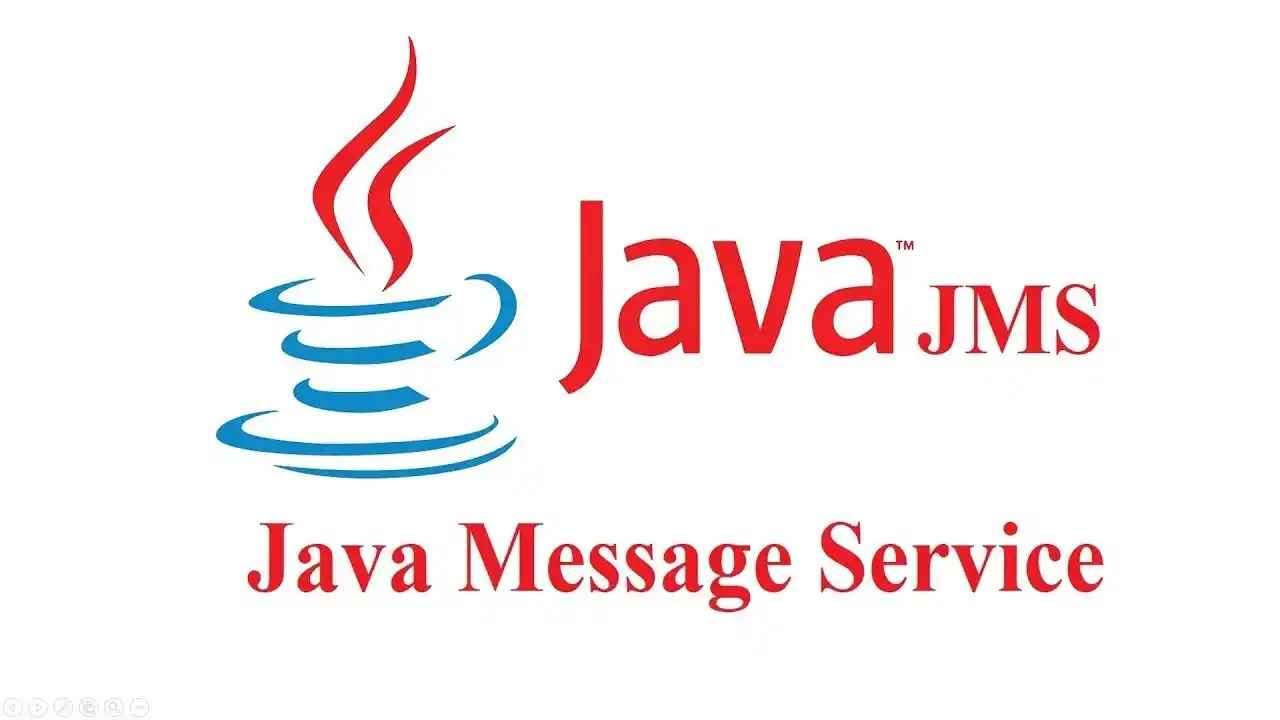
Image Source: google
✉️ JMS Component | 📋 Description |
---|---|
JMS | Java API that allows applications to create, send, receive, and read messages in a loosely coupled way. |
Message | The object that carries data between JMS clients; can be text, object, bytes, or map. |
Producer | Creates and sends messages to a destination (queue or topic). |
Consumer | Receives messages from a destination (queue or topic). |
Queue | A point-to-point messaging model where messages are delivered to one consumer. |
Topic | A publish-subscribe model where messages are broadcast to multiple subscribers. |
ConnectionFactory | Used to create connections with the JMS provider. |
Session | Manages the context for sending and receiving messages. |
RESTful Web Services with JAX-RS
RESTful Web Services are a way to build scalable, stateless web APIs using standard HTTP methods like GET, POST, PUT, and DELETE. JAX-RS (Java API for RESTful Web Services) is a Java framework that simplifies creating RESTful services by providing annotations and tools to map Java classes and methods to web resources.
-
Resource-Based: Uses resources identified by URIs to represent data and services.
-
Annotations: JAX-RS uses annotations like
@Path
,@GET
,@POST
,@PUT
, and@DELETE
to define endpoints and HTTP methods. -
Statelessness: Each request from client to server must contain all information needed to understand and process it, ensuring scalability.
-
Content Negotiation: Supports different data formats like JSON, XML, through built-in message body readers and writers.
-
Integration: Easily integrates with other Java EE technologies and frameworks.
JAX-RS makes implementing RESTful APIs simple and efficient, making it a vital part of Advanced Java Programming Concepts.
Java Authentication and Authorization
🔐 Aspect | Authentication | Authorization |
---|---|---|
Definition | Verifies user identity to confirm who they are. | Determines if the authenticated user has permission to access resources. |
Purpose | To validate credentials like username/password. | To enforce access controls and permissions. |
When It Occurs | Occurs before authorization. | Occurs after successful authentication. |
Key Java Technology | JAAS LoginModule handles authentication. | JAAS Policy and Roles manage authorization. |
Focus | User identity verification. | User access rights and privileges. |
Outcome | User is either authenticated or rejected. | User is granted or denied resource access. |
Design Patterns in Advanced Java
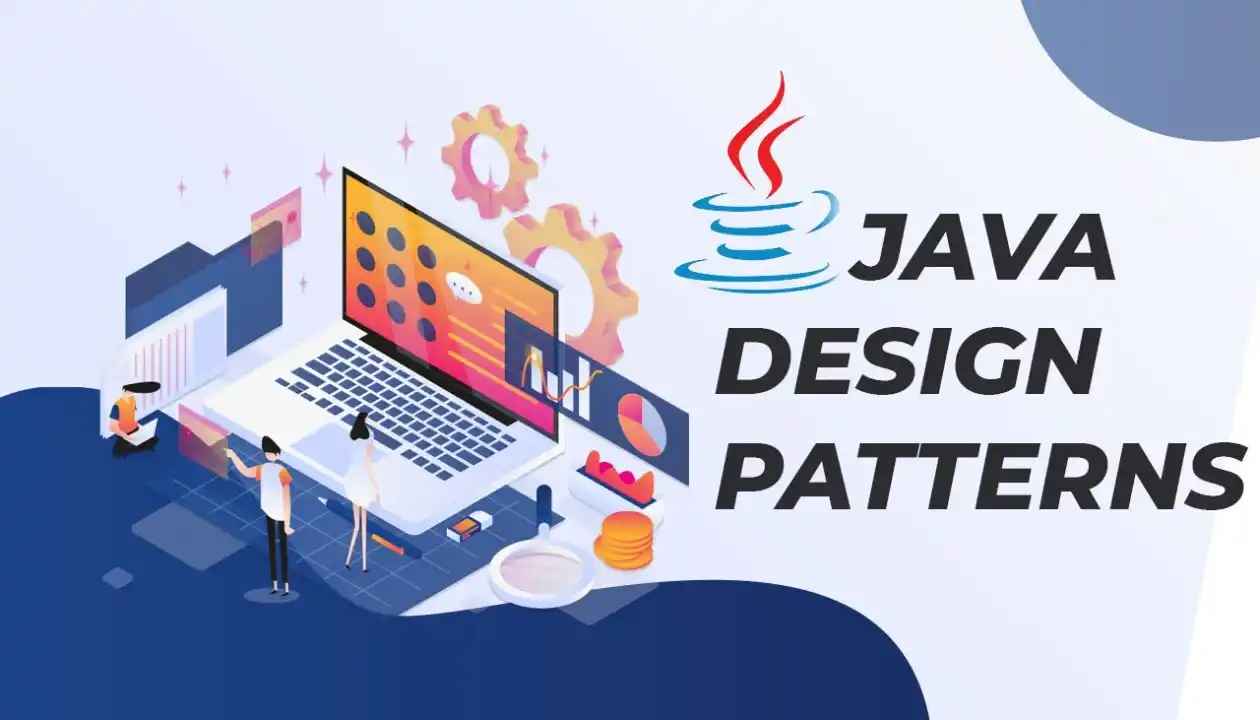
Image Source: google
🎨 Design Pattern | 📋 Description | 🛠️ Usage in Advanced Java |
---|---|---|
Singleton | Ensures a class has only one instance and provides a global point of access. | Used for logging, driver objects, or configuration settings. |
Factory Method | Creates objects without specifying the exact class. | Used in frameworks to instantiate classes dynamically. |
Observer | Defines a one-to-many dependency so when one object changes, all dependents are notified. | Used in event handling and MVC architecture. |
Decorator | Adds responsibilities to objects dynamically without altering their class. | Used for adding features like buffering or encryption. |
DAO (Data Access Object) | Abstracts and encapsulates all access to the data source. | Separates persistence logic from business logic. |
MVC (Model-View-Controller) | Separates application into model, view, and controller layers. | Widely used in web apps using Servlets and JSP. |
Proxy | Provides a placeholder or surrogate for another object to control access. | Used for lazy loading and access control. |
FAQs
Q.1. What is Advanced Java?
A : Advanced Java includes technologies and APIs beyond core Java, like Servlets, JSP, JDBC, and frameworks used to build web and enterprise applications.
Q.2. How is Advanced Java different from Core Java?
A : Core Java covers basic programming concepts, while Advanced Java focuses on building networked, database-driven, and web-based applications.
Q.3. What are common technologies in Advanced Java?
A : Common technologies include Servlets, JSP, JDBC, EJB, JMS, and frameworks like Spring and Hibernate.
Q.4. What is JDBC used for?
A : JDBC connects Java applications to databases to perform SQL operations like insert, update, delete, and query.
Q.5. What is the role of Servlets and JSP?
A : Servlets handle backend logic for web requests; JSP helps create dynamic web pages by embedding Java code in HTML.
Q.6. What is the MVC architecture?
A : MVC separates an application into Model (data), View (UI), and Controller (logic) to improve organization and maintenance.
Q.7. Why is session management important?
A : It tracks user interactions across multiple requests, enabling features like login persistence in web apps.
Q.8. What is Spring Framework?
A : Spring is a popular framework simplifying Java development with features like dependency injection and MVC support.